Update demo to use self-ticking logger
This commit is contained in:
parent
b34d2d7dee
commit
7871b38c80
13
README.md
13
README.md
|
@ -24,28 +24,27 @@ Create a *Historian* and send it *events* to generate an *event log*, where each
|
|||
is tagged with the historian's latest *hash*. Then ensure the order of events was not tampered
|
||||
with by verifying each entry's hash can be generated from the hash in the previous entry:
|
||||
|
||||
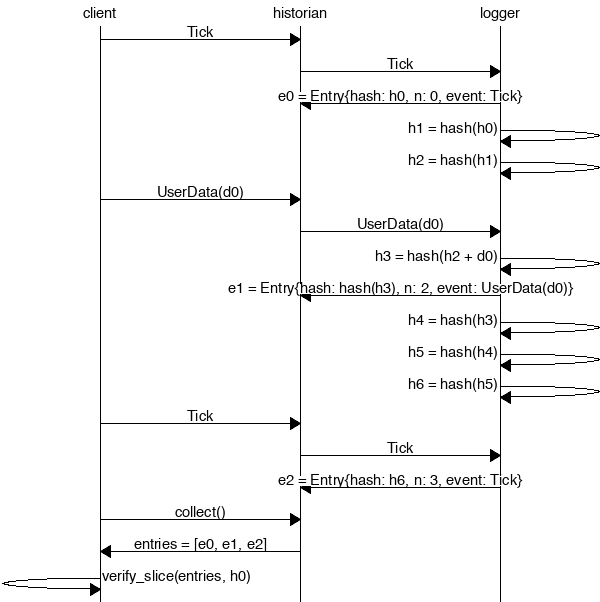
|
||||
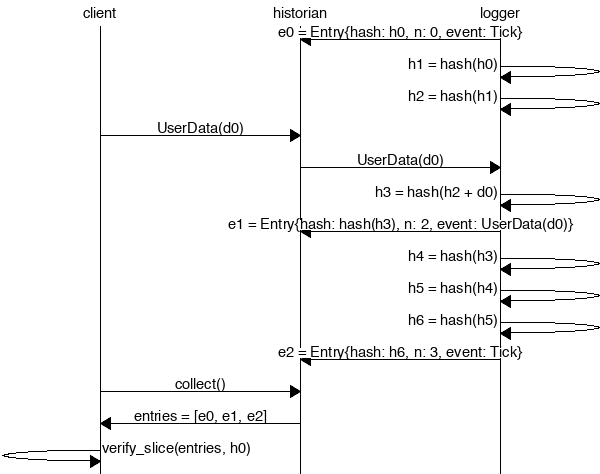
|
||||
|
||||
```rust
|
||||
extern crate silk;
|
||||
|
||||
use silk::historian::Historian;
|
||||
use silk::log::{verify_slice, Entry, Event, Sha256Hash};
|
||||
use std::{thread, time};
|
||||
use std::thread::sleep;
|
||||
use std::time::Duration;
|
||||
use std::sync::mpsc::SendError;
|
||||
|
||||
fn create_log(hist: &Historian) -> Result<(), SendError<Event>> {
|
||||
hist.sender.send(Event::Tick)?;
|
||||
thread::sleep(time::Duration::new(0, 100_000));
|
||||
sleep(Duration::from_millis(15));
|
||||
hist.sender.send(Event::UserDataKey(Sha256Hash::default()))?;
|
||||
thread::sleep(time::Duration::new(0, 100_000));
|
||||
hist.sender.send(Event::Tick)?;
|
||||
sleep(Duration::from_millis(10));
|
||||
Ok(())
|
||||
}
|
||||
|
||||
fn main() {
|
||||
let seed = Sha256Hash::default();
|
||||
let hist = Historian::new(&seed, None);
|
||||
let hist = Historian::new(&seed, Some(10));
|
||||
create_log(&hist).expect("send error");
|
||||
drop(hist.sender);
|
||||
let entries: Vec<Entry> = hist.receiver.iter().collect();
|
||||
|
|
|
@ -1,8 +1,6 @@
|
|||
msc {
|
||||
client,historian,logger;
|
||||
|
||||
client=>historian [ label = "Tick" ] ;
|
||||
historian=>logger [ label = "Tick" ] ;
|
||||
logger=>historian [ label = "e0 = Entry{hash: h0, n: 0, event: Tick}" ] ;
|
||||
logger=>logger [ label = "h1 = hash(h0)" ] ;
|
||||
logger=>logger [ label = "h2 = hash(h1)" ] ;
|
||||
|
@ -13,8 +11,6 @@ msc {
|
|||
logger=>logger [ label = "h4 = hash(h3)" ] ;
|
||||
logger=>logger [ label = "h5 = hash(h4)" ] ;
|
||||
logger=>logger [ label = "h6 = hash(h5)" ] ;
|
||||
client=>historian [ label = "Tick" ] ;
|
||||
historian=>logger [ label = "Tick" ] ;
|
||||
logger=>historian [ label = "e2 = Entry{hash: h6, n: 3, event: Tick}" ] ;
|
||||
client=>historian [ label = "collect()" ] ;
|
||||
historian=>client [ label = "entries = [e0, e1, e2]" ] ;
|
||||
|
|
|
@ -2,21 +2,20 @@ extern crate silk;
|
|||
|
||||
use silk::historian::Historian;
|
||||
use silk::log::{verify_slice, Entry, Event, Sha256Hash};
|
||||
use std::{thread, time};
|
||||
use std::thread::sleep;
|
||||
use std::time::Duration;
|
||||
use std::sync::mpsc::SendError;
|
||||
|
||||
fn create_log(hist: &Historian) -> Result<(), SendError<Event>> {
|
||||
hist.sender.send(Event::Tick)?;
|
||||
thread::sleep(time::Duration::new(0, 100_000));
|
||||
sleep(Duration::from_millis(15));
|
||||
hist.sender.send(Event::UserDataKey(Sha256Hash::default()))?;
|
||||
thread::sleep(time::Duration::new(0, 100_000));
|
||||
hist.sender.send(Event::Tick)?;
|
||||
sleep(Duration::from_millis(10));
|
||||
Ok(())
|
||||
}
|
||||
|
||||
fn main() {
|
||||
let seed = Sha256Hash::default();
|
||||
let hist = Historian::new(&seed, None);
|
||||
let hist = Historian::new(&seed, Some(10));
|
||||
create_log(&hist).expect("send error");
|
||||
drop(hist.sender);
|
||||
let entries: Vec<Entry> = hist.receiver.iter().collect();
|
||||
|
|
|
@ -129,12 +129,11 @@ impl Historian {
|
|||
mod tests {
|
||||
use super::*;
|
||||
use log::*;
|
||||
use std::thread::sleep;
|
||||
use std::time::Duration;
|
||||
|
||||
#[test]
|
||||
fn test_historian() {
|
||||
use std::thread::sleep;
|
||||
use std::time::Duration;
|
||||
|
||||
let zero = Sha256Hash::default();
|
||||
let hist = Historian::new(&zero, None);
|
||||
|
||||
|
@ -168,4 +167,21 @@ mod tests {
|
|||
ExitReason::SendDisconnected
|
||||
);
|
||||
}
|
||||
|
||||
#[test]
|
||||
fn test_ticking_historian() {
|
||||
let zero = Sha256Hash::default();
|
||||
let hist = Historian::new(&zero, Some(2));
|
||||
sleep(Duration::from_millis(3));
|
||||
hist.sender.send(Event::UserDataKey(zero)).unwrap();
|
||||
sleep(Duration::from_millis(1));
|
||||
drop(hist.sender);
|
||||
assert_eq!(
|
||||
hist.thread_hdl.join().unwrap().1,
|
||||
ExitReason::RecvDisconnected
|
||||
);
|
||||
|
||||
let entries: Vec<Entry> = hist.receiver.iter().collect();
|
||||
assert!(verify_slice(&entries, &zero));
|
||||
}
|
||||
}
|
||||
|
|
Loading…
Reference in New Issue